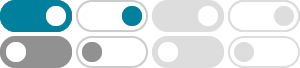
How to Initialize a Dictionary in Python Using For Loop
Jan 22, 2024 · The task of creating a list of dictionaries in Python using a for loop involves iterating over a sequence of values and constructing a dictionary in each iteration. By using a for loop, we can assign values to keys dynamically and append the dictionaries to a list.
Python - Trying to create a dictionary through a for loop
Apr 19, 2017 · You set an item in a dictionary by doing dictionary[key] = item, so in your case you would do: d[count] = i. count+=1. You can use enumerate: d[a] = b. or, using dictionary comprehension: You can do this in one line without using a For loop or a Counter variable:
Python Dictionary with For Loop - GeeksforGeeks
Oct 2, 2023 · In this article, we'll explore Python dictionaries and how to work with them using for loops in Python. In this example, a person is a dictionary with three key-value pairs. The keys are "name," "age," and "city," and the corresponding values are "John," 30, …
Populating a dictionary using two for loops in Python
I'm trying to create a dictionary using two for loops. Here is my code: dicts = {} keys = range(4) values = ["Hi", "I", "am", "John"] for i in keys: for x in values: dicts[i] = x print(dicts) This outputs: {0: 'John', 1: 'John', 2: 'John', 3: 'John'} Why? I was planning on …
python - how to create a new dictionary in for loop ... - Stack Overflow
You want to create a new one for each iteration: new = og_dict.copy() new['salary'] = str(i)+'k' og_dict_list.append(new) print(og_dict_obj) There is a number of ways to copy a dictionary, like just doing dict(of_dict) instead of calling .copy().
How to Create a Dictionary in Python Using a For Loop? - Python …
Feb 18, 2025 · Learn how to create a dictionary in Python using a `for` loop with dictionary comprehension and key-value assignment. This step-by-step guide includes examples.
Python - Loop Dictionaries - W3Schools
Loop Through a Dictionary. You can loop through a dictionary by using a for loop. When looping through a dictionary, the return value are the keys of the dictionary, but there are methods to return the values as well.
Python Dictionary with For Loop - Coding Rooms
Oct 7, 2020 · Using the dictionary structure with for loops is incredibly efficient in python. In this card, I will show you some examples of ways to use dictionaries in for loops. The first example I am going to cover is expanding a dictionary into a list of lists.
Create Dynamic Dictionary using for Loop-Python
Feb 1, 2025 · The task of creating a list of dictionaries in Python using a for loop involves iterating over a sequence of values and constructing a dictionary in each iteration. By using a for loop, we can assign values to keys dynamically and append the dictionaries to a list.
python - Iterating over dictionaries using 'for' loops - Stack Overflow
Jul 21, 2010 · If you want to loop over a dictionary and modify it in iteration (perhaps add/delete a key), in Python 2, it was possible by looping over my_dict.keys(). In Python 3, the iteration has to be over an explicit copy of the keys (otherwise it throws a RuntimeError ) because my_dict.keys() returns a view of the dictionary keys, so any change to my ...