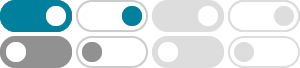
Printing a Tree data structure in Python - Stack Overflow
def __init__(self, value, children = []): self.value = value. self.children = children. def __repr__(self, level=0): ret = "\t"*level+repr(self.value)+"\n" for child in self.children: ret += child.__repr__(level+1) return ret. This code prints the tree in the following way: 'daughter' 'granddaughter' 'grandson' 'son' 'granddaughter' 'grandson'
Binary Tree in Python - GeeksforGeeks
Feb 27, 2025 · A Binary search tree is a binary tree where the values of the left sub-tree are less than the root node and the values of the right sub-tree are greater than the value of the root node. In this article, we will discuss the binary search tree in Python.
How can I implement a tree in Python? - Stack Overflow
Mar 1, 2010 · For example, a binary tree might be: def __init__(self): self.left = None. self.right = None. self.data = None. You can use it like this: If you need an arbitrary number of children per node, then use a list of children: def __init__(self, data): self.children = [] self.data = data.
representing a tree as a list in python - Stack Overflow
Aug 25, 2016 · I'm learning python and I'm curious about how people choose to store (binary) trees in python. Is there something wrong in storing the nodes of the tree as a list in python? something like: [0,1,2,3,4,5,6,7,8]
Python Code to Print a Binary Tree - Python Guides
Jul 13, 2023 · In this tutorial, we will create a binary tree in Python and complete Python code to print a binary tree. Firstly, we need to create the binary tree structure. Here, we’ll define a simple Node class: def __init__(self, data): self.data = data. self.left = None. self.right = None.
Tree Data Structure in Python - PythonForBeginners.com
Jun 9, 2023 · A Python tree is a data structure in which data items are connected using references in a hierarchical manner in the form of edges and nodes. Each tree consists of a root node from which we can access the elements of the tree.
Tree in Python - Sanfoundry
The following section contains Python programs on trees, binary trees, binary search trees, binomial tree, nodes of a tree, tree traversals, BFS, and DFS tree traversals. Each sample program includes a program description, Python code, and program output.
Python Tree Construction and Manipulation - Online Tutorials …
Apr 15, 2021 · Learn how to construct a tree in Python and perform insertion, deletion, and display operations with this comprehensive tutorial.
5 Best Ways to Construct and Manage a Tree in Python
Mar 7, 2024 · We aim for a tree where nodes can be added (as children), removed, and displayed, reflecting updates accurately. An Object-Oriented Programming (OOP) approach in Python allows for the creation of a tree structure by defining a Node class with attributes for data storage and pointers to child nodes.
How to Print Binary Tree in Python - Delft Stack
Feb 2, 2024 · Print the Whole Binary Tree Using Python. Here is the entire Binary Tree. We can run it and learn more about how a binary tree is printed using Python with the random library of Python.