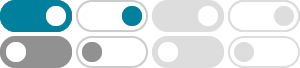
python - Reverse a string without using reversed() or [::-1]?
Sep 9, 2013 · You can simply reverse iterate your string starting from the last character. With python you can use list comprehension to construct the list of characters in reverse order and then join them to get the reversed string in a one-liner: def reverse(s): return "".join([s[-i-1] for i …
Reverse string without using function in Python - CodeSpeedy
How to reverse a string in python without using functions. Use of for loop for iterating the string value and concatenating it with an empty string.
How to Reverse a String in Python Without Using Slicing or …
Mar 2, 2025 · The typical way to reverse a string in Python is using slicing ([::-1]) or built-in functions (reversed() and join()). However, to reverse a string in Python without using slicing or built-in functions, we need to manually iterate over the string and construct the reversed version.
How to reverse a String in Python - GeeksforGeeks
Nov 21, 2024 · The slicing method (s[::-1]) is the most concise and efficient way to reverse a string. The reversed() function is easy to use and maintains readability. Looping & List comprehension provides more control over the reversal process.
Reverse a Python string without omitting start and end slice
Mar 28, 2015 · The notation string[begin:end:increment] can be described with the following Python program: def myreverse( string, begin, end, increment ): newstring = "" if begin >= 0 and end < 0: begin = -1 for i in xrange(begin, end, increment): try: newstring += string[i] except IndexError: pass return newstring
reversing a string in python without using methods or slicing
Mar 5, 2015 · This function tries to reverse a provided string. z= [] x=range (10) def reve (y): for i in range (len (y)): z.append (y [len-1-i]) return z print reve (x) This is the error I got. Traceb...
How to reverse a string in Python without slicing
If you want to reverse a string in Python without using slicing, you can use a loop to iterate through the characters of the string and construct the reversed string. Here’s an example using a for loop: def reverse_string(input_string): reversed_string = "" for char in input_string: reversed_string = char + reversed_string return reversed ...
Reverse a String in Python Without using inbuilt Function - Know Program
We will develop a program to reverse a string in python without using inbuilt function. We read a string and reverse a string using slice operations. Syntax of slicing operation:- string[::-1]
Reverse String in Python Using 5 Different Methods - STechies
Mar 3, 2020 · In this article you will learn 5 different ways to reverse the string in Python such as using for loop, Using while loop, Using slicing, Using join() and reversed() and using list reverse(). In python string library, there is no in-build “reverse” function to reverse a string, but there are many different ways to reverse a string.
How to Reverse a String in Python? - Python Guides
Sep 11, 2024 · To reverse a string in Python using slicing, you can use the concise syntax reversed_string = original_string[::-1]. This method works by starting at the end of the string and moving backward with a step of -1, effectively reversing the string.