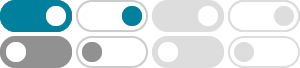
Read a single column of a CSV and store in an array
Jan 12, 2014 · One option is just to read in the entire csv, then select a column: data = pd.read_csv("data.csv") data['title'] # as a Series data['title'].values # as a numpy array As @dawg suggests, you can use the usecols argument, if you also use the squeeze argument to avoid some hackery flattening the values array...
Store numpy.array in cells of a Pandas.DataFrame
Aug 7, 2017 · Preset the type of your column to object, this will allow you to store a NumPy array as-is: df['COL_ARRAY'] = pd.Series(dtype='object') df['COL_ARRAY'] = df.apply(lambda r: np.array(do_something_with_r), axis=1)
How to Retrieve an Entire Row or Column of an Array in Python?
Nov 12, 2020 · In this article, we will cover how to extract a particular column from a 1-D array of tuples in python. Example Input: [(18.18,2.27,3.23),(36.43,34.24,6.6),(5.25,6.16,7.7),(7.37,28.8,8.9)] Output: [3.23, 6.6 , 7.7 , 8.9 ] Explanation: Extracting the 3rd column from 1D array of tuples.
Python: How to read file and store certain columns in array
Jan 4, 2016 · You could do that with pandas using read_table to read your data, iloc to subset your data, values to get values from DataFrame and tolist method to convert numpy array to list:
How to Convert a Dataframe Column to Numpy Array
Feb 3, 2024 · Converting a NumPy array into a Pandas DataFrame makes our data easier to understand and work with by adding names to rows and columns and giving us tools to clean and organize it. In this article, we will take a look at methods to convert a …
How to access a NumPy array by column - GeeksforGeeks
Apr 23, 2023 · Access the i th column of a Numpy array using transpose. Transpose of the given array using the .T property and pass the index as a slicing index to print the array.
Python Arrays - W3Schools
Arrays are used to store multiple values in one single variable: Create an array containing car names: What is an Array? An array is a special variable, which can hold more than one value at a time. If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
csv to array python
Jan 1, 2016 · For more intuitive data access, it’s beneficial to store CSV columns in separate arrays. csvReader = csv.reader(csvDataFile) dates.append(row[0]) scores.append(row[1]) If you have multiple CSV files with identical formats, encapsulate the reading logic in a function to avoid code duplication. dates = [] scores = []
Arrays In Python: The Complete Guide With Practical Examples
Arrays are one of the fundamental data structures in programming, and Python offers several ways to work with them. When I first started working with Python more than a decade ago, understanding arrays was a game-changer for handling collections of data efficiently.
Working with CSV Columns in Python Arrays - CodeRivers
Feb 28, 2025 · This blog post will explore how to put columns of a CSV file into arrays in Python, covering fundamental concepts, usage methods, common practices, and best practices. In data analysis and manipulation tasks, working with Comma - Separated Values (CSV) files is a common practice.
- Some results have been removed