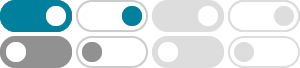
Reverse a String in Java - GeeksforGeeks
Mar 27, 2025 · We can use character array to reverse a string. Follow Steps mentioned below: First, convert String to character array by using the built-in Java String class method toCharArray(). Then, scan the string from end to start, and print the character one by one.
java - How do I reverse a String array? - Stack Overflow
Nov 7, 2014 · First, you could use Arrays.toString(Object[]) to print your String[]. Then you can iterate half of the array and swap the String at the current position with it's corresponding pair (at the length - current index, remembering that Java arrays are zero indexed so the last position in an array is array.length - 1). Finally, print again. Like,
String array reverse Java - Stack Overflow
Mar 9, 2014 · I am trying to reverse all the strings in an array in java, but seem to over write all of them with the first. int flag=0; String reverse; for(int i=0;i<n;i++) // n is declared globally as number of strings. reverse=""; for (int j=s[i].length()-1;i>=0;i--) reverse=reverse+s[i].charAt(j); if(s[i].equals(reverse)) System.out.println(s[i]); .
Reverse String Using Array in Java - Tpoint Tech
Mar 21, 2025 · To reverse a string, the easiest technique is to convert it to a character array and then swap the characters at both ends of the array. In Java, you can do the following: The original String is Hello World! Reversed String is !dlroW olleH. The toCharArray () method converts the input string into a character array.
java - How do I reverse every string in a string array ... - Stack Overflow
May 19, 2020 · Simply iterate the array, and replace each entry with its reverse. You can use a StringBuilder to reverse a String, calling StringBuilder.reverse. Like, public static void reverse(String[] array) { for (int i = 0; i < array.length; i++) { array[i] = new StringBuilder(array[i]).reverse().toString(); } } And then to test it
Reverse A String In Java – 4 Ways | Programs - Java Tutoring
Mar 2, 2025 · Java Code Reverse A String – Using Array 1) We are using a character array to reverse the given string. 2) Read the entered string using scanner object scan.nextLine() and store it in the variable str.
Reverse a String in Java in 10 different ways | Techie Delight
Apr 1, 2024 · This post covers 10 different ways to reverse a string in java by using StringBuilder, StringBuffer, Stack data structure, Java Collections framework reverse() method, character array, byte array, + (string concatenation) operator, Unicode right-to-left override (RLO) character, recursion, and substring() function.
How to Reverse a String in Java Using Different Methods
Apr 9, 2025 · String reversal is a fundamental problem frequently asked in coding interviews. we will explore multiple approaches to reverse a string in Java, with step-by-step explanations and practical code examples. 1. Using StringBuilder Java provides StringBuilder, which has a built-in reverse() method to easily reverse a string. public class ReverseStringExample { public static void … How to Reverse ...
Different Ways to Reverse a String in Java - CodeGym
Jan 31, 2023 · This short article will guide you through various ways by which we can reverse a string in Java such as recursion, array, etc.
How to Reverse a String in Java:13 Best Methods - Simplilearn
Apr 14, 2025 · You can use Collections.reverse() to reverse a Java string. Use these steps: Create an empty ArrayList of characters, then initialize it with the characters of the given string with String.toCharArray().