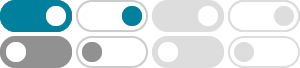
JavaScript Array reduce() Method - W3Schools
The reduce() method executes a reducer function for array element. The reduce() method returns a single value: the function's accumulated result. The reduce() method does not execute the function for empty array elements.
JavaScript ES5 - W3Schools
ES5 lets you define object methods with a syntax that looks like getting or setting a property. This example creates a getter for a property called fullName:
JavaScript toFixed() Method - W3Schools
The toFixed() method converts a number to a string. The toFixed() method rounds the string to a specified number of decimals.
JavaScript Array Methods - W3Schools
The slice() method creates a new array. The slice() method does not remove any elements from the source array.
W3Schools Tryit Editor
The W3Schools online code editor allows you to edit code and view the result in your browser
Javascript ES6 - W3Schools
const myPromise = new Promise(function(myResolve, myReject) { setTimeout(function() { myResolve("I love You !!"); }, 3000);}); myPromise.then(function(value) { document.getElementById("demo").innerHTML = value;});
JavaScript Array Iteration - W3Schools
The reduce() method runs a function on each array element to produce (reduce it to) a single value. The reduce() method works from left-to-right in the array. See also reduceRight() .
JavaScript Array map() Method - W3Schools
map() creates a new array from calling a function for every array element. map() does not execute the function for empty elements. map() does not change the original array.
PHP array_reduce() Function - W3Schools
The array_reduce() function sends the values in an array to a user-defined function, and returns a string. Note: If the array is empty and initial is not passed, this function returns NULL.
JavaScript Array entries() Method - W3Schools
The entries() method returns an Iterator object with the key/value pairs from an array: [0, "Banana"] [1, "Orange"] [2, "Apple"] [3, "Mango"] The entries() method does not change the original array.