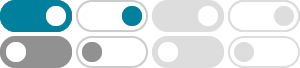
Getting random numbers in Java - Stack Overflow
May 5, 2011 · The first solution is to use the java.util.Random class: import java.util.Random; Random rand = new Random(); // Obtain a number between [0 - 49]. int n = rand.nextInt(50); // Add 1 to the result to get a number from the required range // (i.e., [1 - 50]). n += 1; Another solution is using Math.random(): double random = Math.random() * 49 + 1 ...
How do I generate random integers within a specific range in Java ...
The Java Math library function Math.random() generates a double value in the range [0,1). Notice this range does not include the 1. In order to get a specific range of values first, you need to multiply by the magnitude of the range of values you want covered. Math.random() * ( Max - Min )
Java Generate Random Number Between Two Given Values
Mar 11, 2011 · Java doesn't have a Random generator between two values in the same way that Python does. It actually only takes one value in to generate the Random. What you need to do, then, is add ONE CERTAIN NUMBER to the number generated, which will cause the number to be within a range.
How Java random generator works? - Stack Overflow
Feb 17, 2016 · If you use Random, then No. Not for any JVM on any OS. The sequence produced by an LCNG is definitely not random, and has statistical properties that are significantly different from a true random sequence. (The sequence will be strongly auto-correlated, and this will show up if you plot the results of successive calls to Random.nextInt().)
java - How to randomly pick an element from an array - Stack …
I wouldn't create Random() each time you run the function: the random generator is supposed to have history. If it haven't, it's extremely predictable. If it haven't, it's extremely predictable. It's not a problem at all in this case — but it should be mentioned that array[(int)(System.currentTimeMillis() % array.length)] is just as good as ...
Java random number with given length - Stack Overflow
Mar 22, 2011 · random_float() is a pseudo-code function (i.e. it does not exist) that returns a random floating-point number between 0 (inclusive) and 1 (exclusive). – Bombe Commented Mar 22, 2011 at 14:26
Random numbers with Math.random() in Java - Stack Overflow
Oct 27, 2011 · Math.random() Returns a double value with a positive sign, greater than or equal to 0.0 and less than 1.0. Now it depends on what you want to accomplish.
How does Math.random() EXACTLY work in Java? - Stack Overflow
Dec 15, 2017 · Math.random() It generates a random number between 0.0 to 1.0(always less then 1.0).So,in your problem as you said that you want to generate random number between 1 to 6 that is why you have multiplied it by 6, so that any random number the function will return will be multiplied by 6 and then + 1 ,so that it always stay between 1-6 .
How would you give random limits in java? - Stack Overflow
Jan 18, 2012 · The answers provided here are correct if you are looking for an integer. However, if you are not looking for an integer random number, I think the below solution would work. If you want a random number between 50 and 100, use this: randomNumber = 50+(Math.random()*50);
how does random () actually work? - Stack Overflow
Jan 13, 2012 · random() is a so called pseudorandom number generator (PRNG). random() is mostly implemented as a Linear congruential generator. This is a function of the form X(n+1) (aXn +c) modulo m. Xn is the sequence of generated pseudorandom numbers. The genarated sequence of numbers is easy guessable.