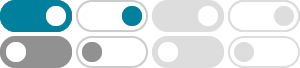
Python Programs to Print Patterns – Print Number, Pyramid, Star ...
Sep 3, 2024 · In this lesson, you’ll learn how to print patterns using the for loop, while loop, and the range() function. This article teaches you how to print the following patterns in Python.
Printing Pyramid Patterns in Python - GeeksforGeeks
Mar 3, 2025 · Exploring and creating pyramid patterns in Python is an excellent way to enhance your programming skills and gain a deeper understanding of loops and control structures. By modifying and experimenting with the provided examples, you can create a variety of captivating patterns using Python.
Python | Print an Inverted Star Pattern - GeeksforGeeks
Aug 24, 2023 · An inverted star pattern involves printing a series of lines, each consisting of stars (*) that are arranged in a decreasing order. Here we are going to print inverted star patterns of desired sizes in Python. Examples: 1) Below is the inverted star pattern of size n=5 (Because there are 5 horizontal lines or rows consist of stars
Python Program to Create Pyramid Patterns
In this example, you will learn to print half pyramids, inverted pyramids, full pyramids, inverted full pyramids, Pascal's triangle, and Floyd's triangle in Python Programming.
5 Best Ways to Create Python Program to Print an Inverted Star Pattern
Mar 7, 2024 · Method 1: Using Nested For Loops. This method involves using two for loops: the outer loop to handle the number of lines and the inner loop for printing stars and managing spaces. It’s one of the most straightforward implementations for pattern generation in Python and is very beginner-friendly. Here’s an example:
python - Program for an upright and inverted triangle pattern …
Nov 4, 2023 · I am new to Python and wanted to create a program which prints this star pattern using one for loop and an if-else statement: * ** *** **** ***** **** *** ** * What I've done so far: stars = "*" for i in range (0,10): if i <5: print(stars) stars = stars + …
Python Program to print an Inverted Star Pattern - Studytonight
Aug 23, 2021 · We utilize the built-in function input to get the number of rows in an inverted star pattern from the user (). Because the input () method returns a string value, we must convert the provided number to an integer using the int () function (). Then, using a loop, we create an inverse pyramid pattern. The patterns are as follows:
How to print a Triangle Pyramid pattern using a for loop in Python ...
Here's the easiest way to print this pattern: print(" "*(n-i) + "* "*i) You have to print spaces (n-i) times, where n is the number of rows and i is for the loop variable, and add it to the stars i times. Try this: k = 2*n - 2. for i in range(0, n): . for j in range(0, k): .
Python Program to Print Inverted Triangle Numbers Pattern
Write a Python program to print an inverted triangle numbers pattern using nested for loop range with an example. for j in range(1, i): print(end = ' ') for k in range(1, rows - i + 2): print(k, end = ' ') print() This example displays the inverted triangle pattern of numbers using a while loop. j = 1. while(j < i): print(end = ' ') j = j + 1.
Reverse Pyramid Pattern in Python
Mar 30, 2023 · Python Program to print a Reverse Pyramid Pattern or Reverse Triangle Pattern. Run 3 for loops, one main for row looping, and the other 2 sub-loops for column looping, in the first loop, loop through the range of the triangle for row looping. That is it for this tutorial.