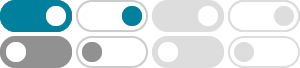
Print the Fibonacci sequence – Python | GeeksforGeeks
Mar 22, 2025 · To print the Fibonacci sequence in Python, we need to generate a series of numbers where each number is the sum of the two preceding ones, starting from 0 and 1. The Fibonacci sequence follows a specific pattern that begins with 0 and 1, and every subsequent number is the sum of the two previous numbers.
How do I create a module for a fibonacci sequence in Python on …
Jan 22, 2015 · # Fibonacci numbers module def fib(n): # write Fibonacci series up to n a, b = 0, 1 while b < n: print(b, end=' ') a, b = b, a+b print() def fib2(n): # return Fibonacci series up to n result = [] a, b = 0, 1 while b < n: result.append(b) a, b = b, a+b return result
Program to define a module to find Fibonacci Numbers and …
Nov 22, 2024 · # fib.py - module # Function to return the nth Fibonacci number def fibonacci(n): if n <= 0: return 0 elif n == 1: return 1 else: return fibonacci(n - 1) + fibonacci(n - 2)
write a python program to define a module to find fibonacci numbers …
Apr 1, 2023 · In this article you will learn a Python program to define a module to find the fibonacci series and fibonacci of a number and then use this module in your program. Let's try to learn these two variants of the Python program.
Python Program to Print the Fibonacci sequence
Write a function to get the Fibonacci sequence less than a given number. The Fibonacci sequence starts with 0 and 1 . Each subsequent number is the sum of the previous two.
Fibonacci Series Program in Python - Python Guides
Aug 27, 2024 · In this Python tutorial, we covered several methods to generate the Fibonacci series in Python, including using for loops, while loops, functions, recursion, and dynamic programming. We also demonstrated how to generate the Fibonacci series up to a specific range without using recursion.
Write a python program to define a module to find fibonacci numbers …
Feb 5, 2024 · To define a module to find Fibonacci numbers in Python, you can follow these steps: Create a new Python file and save it with a .py extension, such as fibonacci_module.py. In the fibonacci_module.py file, define a function to find the Fibonacci numbers.
Write A Python Program For Fibonacci Series (3 Methods + Code)
The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding numbers. In Python, you can generate the Fibonacci series using a loop or recursion. For example, the Fibonacci series up to 10 terms would be: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34].
Fibonacci Numbers in Python: A Comprehensive Guide
Jan 26, 2025 · In this blog, we will explore how to work with Fibonacci numbers in Python, covering fundamental concepts, different usage methods, common practices, and best practices. The Fibonacci sequence is defined by the recurrence relation: [ F (n) = F (n - 1) + F (n - 2) ] where ( F (0) = 0 ), ( F (1) = 1 ), and ( n \gt 1 ).
py-fibonacci - PyPI
Jul 5, 2020 · Fibonacci Series is a list of numbers in which one number on the series is the sum of the previous two numbers. ... e.g. fibonacci (39) -> returns a list, you may assign it to a variable or print it. With an 'end' number argument:: so it is possible to list the fibonacci series up to that number and start (default: 0) number argument is optional.