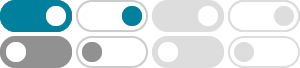
Jun 4, 2021 · For Example with Range Exercise: Find and print all of the positive integers less than or equal to 100 that are divisible by both 2 and 3, using a for loop. def main(): """ Print integers in [1..100] divisible by both 2 and 3. """ for num in range(1, 101):
Similarly, an if...elif structure need not have an else In Python: if condition1: statements1 elif condition2: statements2 Write a Python script to implement the following pseudocode: Prompt user to input an integer, store as x If x < 5, print “The number is less than 5.” Else, if x < 10, print “The number is between 5 and 10.”
A common way to manipulate a sequence is to perform some action for each element in the sequence. This is called looping or iterating over the elements of a sequence.
Two kinds of Loops in Python while loops The evaluation of a boolean expression determines when the repetition stops Changes in values of variables lead to different evaluations of the boolean expression on each repetition When the expression is evaluated as False, the loop halts If the expression can never evaluate as False, the loop is endless
In this chapter, you will learn about loop statements in Python, as well as techniques for writing programs that simulate activities in the real world. Drawing tiles... A loop executes instructions repeatedly while a condition is True. The loop body will only execute if the test condition is True. • Which version will execute the loop body?
Lecture_11 - Purdue University
Loops allow repeated execution of the same set of statements on all the objects within a sequence. Using an index based for loop is best suited for making changes to items within a list.
In Python a for-loop is used to step through a sequence e.g., count through a series of numbers or step through the lines in a file. for i in range (1, 4, 1): print("i=", i) print("Done!") for i in range (3, …
Informatics-Practices-Looping-and-Lists | PDF | Control Flow | Python …
This document serves as a comprehensive guide to using loops and lists in Python, detailing their syntax, practical examples, and applications. It covers both 'for' and 'while' loops, nested loops, list creation and manipulation, and list comprehension.
Python has a function (generator) enumerate. It produces pairs of item index and the index itself: In [12]: list(enumerate(workdays)) Out[12]: [(0, 'Monday'), (1, 'Tuesday'), (2, 'Wednesday'), (3, 'Thursday'), (4, 'Friday')] We can use it in the for-loop as follows. [13]: for i, day_name in enumerate(workdays): print('The day nr.', i, 'is', day ...
Python programming language provides following types of loops to handle looping requirements. Click the following links to check their detail. Repeats a statement or group of statements while a given condition is true. It tests the condition before executing the loop body.
- Some results have been removed