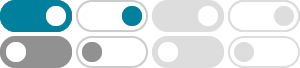
JavaScript Program to Check Whether a String is Palindrome or Not
Write a function to find whether a string is palindrome. A palindrome is a string that reads the same forwards and backwards.
Palindrome in JavaScript - GeeksforGeeks
Aug 21, 2024 · We will understand how to check whether a given value is a palindrome or not in JavaScript. A palindrome is a word, phrase, number, or any sequence that reads the same forward and backward. For instance, “madam” and “121” are palindromes. To perform this check we will use the following approaches:
How to check whether a passed string is palindrome or not in JavaScript ...
Jul 10, 2024 · Below are the methods by which we can check whether a passed string is palindrome or not: First, we iterate over a string in forward and backward directions. Check if all forward and backward character matches, and return true. If all forward and backward character does not matches, return false. If a return is true, it is a palindrome.
Two Ways to Check for Palindromes in JavaScript
Mar 22, 2016 · Return true if the given string is a palindrome. Otherwise, return false. A palindrome is a word or sentence that’s spelled the same way both forward and backward, ignoring punctuation, case, and spacing.
JavaScript Program to Check if a String is a Palindrome - Java …
This program will help you check whether a given string is a palindrome using JavaScript. Problem Statement. Create a JavaScript program that: Accepts a string. Checks if the string is a palindrome. Returns true if the string is a palindrome and false otherwise. Example: Input: "madam" Output: madam is a palindrome. Input: "hello"
How to check the given string is palindrome using JavaScript
Mar 22, 2023 · How to Check if a String is Palindrome or Not Without Filters in VueJS ? In VueJS, we can create a simple palindrome checking function by comparing the characters at the proper corresponding positions in the string.
Check if a String is a Palindrome in JavaScript
Jun 27, 2015 · I am working on a function to check if a given string is a palindrome. My code seems to work for simple one-word palindromes but not for palindromes that feature capitalization or spaces. function palindrome(str) { var palin = str.split("").reverse().join(""); if (palin === str){ return true; } else { return false; } } palindrome("eye ...
JavaScript: Check whether a passed string is palindrome or not …
Feb 28, 2025 · Write a JavaScript function that verifies whether a number is a palindrome without converting the number to a string. Write a JavaScript function that determines if an array of characters forms a palindrome. Write a JavaScript function that checks for a palindrome using a recursive approach.
Check Whether a String is Palindrome or Not in JavaScript
Checking whether a string is a palindrome is a common problem in programming and can be approached in various ways. This guide will walk you through different methods to determine if a given string is a palindrome using JavaScript. Create a JavaScript program that: Accepts a string input. Determines whether the string is a palindrome.
JavaScript Program to Check Whether a String is Palindrome or Not
Nov 12, 2024 · In this article, you will learn how to check whether a string is a palindrome using JavaScript. You'll explore multiple examples that demonstrate how to implement this function and discuss improvements to handle edge cases effectively.
- Some results have been removed