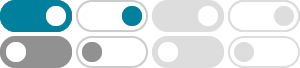
How can I test if a letter in a string is uppercase or lowercase …
Jun 22, 2009 · You're basically checking if the character code of the letter comes before [. Since the character codes for Z, [, a are 90, 91, 97 respectively, the comparison is truthy for uppercase letters and falsy for lowercase letters.
javascript - Check if user input string contains only uppercase and…
May 10, 2017 · I ultimately want to check to make sure the user has input only uppercase or lowercase characters (no numbers, special characters, spaces, etc.). If any unacceptable characters are found, it alerts the user of invalid input. Otherwise, the program proceeds as normal and no error/alert is thrown. Below is the JavaScript code I have currently.
Check if Letter in String is Uppercase or Lowercase in JS
To check if a letter in a string is uppercase or lowercase: Use the toUpperCase() method to convert the letter to uppercase. Compare the letter to itself. If the comparison returns true, the letter is uppercase, otherwise, it's lowercase. We used the String.toUpperCase () method to convert the character to uppercase so we can compare it.
JavaScript Program to Validate String for Uppercase
Jul 16, 2024 · Example: In this example The function checkString analyzes a string for uppercase, lowercase, numeric, and special characters, returning an object indicating their presence. It’s then tested with a sample string.
javascript : validate upper case and lower case - Stack Overflow
May 1, 2014 · how to write regular expression for 1st letter upper case and remaining are lower case for validation in javascript
Top 3 Methods to Determine Uppercase and Lowercase Letters
Nov 23, 2024 · How can I effectively determine if a letter in a string is uppercase or lowercase using JavaScript? This query tackles a common programming task that can be addressed through various techniques. The first approach leverages simple character comparison using the built-in string methods toUpperCase() and toLowerCase().
JavaScript String big() Method - W3Schools
The big() method returns a string embedded in a <big> tag: <big>string</big> The <big> tag is not supported in HTML5.
Testing Uppercase and Lowercase Letters in JavaScript: Methods and Examples
JavaScript provides two fundamental methods to test the case of a letter: toUpperCase() and toLowerCase(). The toUpperCase() method converts a letter to uppercase, while the toLowerCase() method converts it to lowercase.
How to Check for At Least One Capital Letter in JavaScript
Checking for at least one capital letter in a string is simple yet crucial in JavaScript. It’s used in many places like password checks, form input checks, and data processing. Regular Expressions (Regex) make this task easy and quick with just a few lines of code.
How do I make an input field accept only letters in javaScript?
If you want only letters - so from a to z, lower case or upper case, excluding everything else (numbers, blank spaces, symbols), you can modify your function like this: if (document.myForm.name.value == "") { alert("Enter a name"); document.myForm.name.focus(); return false; if (!/^[a-zA-Z]*$/g.test(document.myForm.name.value)) {