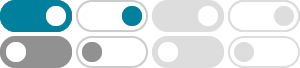
Are infinite for loops possible in Python? - Stack Overflow
The quintessential example of an infinite loop in Python is: while True: pass To apply this to a for loop, use a generator (simplest form): def infinity(): while True: yield This can be used as follows: for _ in infinity(): pass
python - Easy way to keep counting up infinitely - Stack Overflow
Jul 11, 2012 · Take a look at itertools.count (). From the docs: Make an iterator that returns evenly spaced values starting with n. Equivalent to: # count(10) --> 10 11 12 13 14 ... # count(2.5, 0.5) -> 2.5 3.0 3.5 ... n = start. while True: yield n. n += step. So for example: print(i) would generate an infinite sequence starting with 13, in steps of +1.
Infinite loops using 'for' in Python - Stack Overflow
Jan 11, 2018 · For loops are iterating through elements of a generator. You can write an infinite generator using the yield keyword though. range is a class, and using in like e.g. range(1, a) creates an object of that class. This object is created only once, it …
Create an infinite loop in Python - CodeSpeedy
You can create an infinite loop through any method (for or while) by not writing the termination condition or making a termination condition so the loop can never achieve it. For example, in a for loop , you can set the termination condition if the iterator becomes 2.
Python while loop (infinite loop, break, continue, and more)
Aug 18, 2023 · Basic syntax of while loops in Python; Break a while loop: break; Continue to the next iteration: continue; Execute code after normal termination: else; Infinite loop with while statement: while True: Stop the infinite loop using keyboard interrupt (ctrl + c) Forced termination
Python Infinite While Loop - Tutorial Kart
To write an Infinite While Loop in Python, we have to make sure that the condition always evaluates to true. In this tutorial, we learn some of the ways to write an inifinte while loop in Python.
Python Tutorial: How to Create Infinite Loops in Python?
Oct 21, 2024 · In Python, the most common way to create an infinite loop is by using the while statement. The syntax for a while loop is as follows: # code to execute. To create an infinite loop, you can set the condition to True. Here’s a simple example: print("This loop will run forever!")
Python - Infinite While loop - Python Examples
In Python, an infinite While loop is a While loop where the condition is always evaluated to True, and the While loop is run indefinitely. In this tutorial, you shall learn how to define an infinite While loop with examples.
Over-Engineering the Infinite Loop in Python - Medium
Aug 25, 2022 · In this article, I’ll show you seven ways of achieving an infinite loop in Python, starting from the most common approach and gradually moving toward more complicated and exotic techniques.
loops - Looping from 1 to infinity in Python - Stack Overflow
Jun 27, 2014 · If you want to use a for loop, it's possible to combine built-in functions iter (see also this answer) and enumerate for an infinite for loop which has a counter. We're using iter to create an infinite iterator and enumerate provides the counting loop variable.