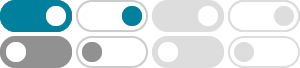
How to Perform Multiplication in Python? - AskPython
Jun 30, 2021 · The most simple one is using the asterisk operator(*), i.e., you pass two numbers and just printing num1 * num2 will give you the desired output. This tutorial will guide you through the different ways to do multiplication in Python.
How to Multiply in Python? [With Examples] - Python Guides
Aug 8, 2024 · To multiply two numbers in Python, you simply use the * operator. For example, result = 5 * 3 will yield 15 . This method works for integers, floats, and even complex numbers, making it a versatile and straightforward way to perform multiplication in Python.
Python Program for Multiplication of Two Numbers
# Python program to multiply two number # take inputs num1 = float(input('Enter first number: ')) num2 = float(input('Enter second number: ')) # calculate product product = num1*num2 # print multiplication value print("The Product of Number: %0.2f" %product)
Python Program to Multiply Two numbers - Tutorial Gateway
Write a Python program to multiply two numbers. This example accepts two integer values and calculates the product of those two numbers. num1 = int(input("Please Enter the First Number to Multiply = ")) num2 = int(input("Please Enter the second Number to Multiply = ")) mul = num1 * num2 print('The Result of Multipling {0} and {1} = {2}'.format ...
Python Program to multiply Two Numbers Without using
We will develop a python program to multiply two numbers without using * operator. We will give two numbers num1 and num2. Python programs will multiply these numbers using a For Loop. We will also develop a python program to multiply two numbers using recursion. Mathematically, We will take two numbers while declaring the variables.
Python Program to Multiply Two Numbers using Function
Multiplication of two numbers in python using multiplication operator (*). Also, we will calculate the product of two numbers using recursion. Mathematically, This is the simplest and easiest way to multiply two numbers in Python.
Multiplication - Python Examples
In this example, we shall take two integers, multiply them, and print the result. print(result) You can multiply more than two numbers in a single statement. This is because, Multiplication Operator supports chaining. print(result) Float is one of the numeric datatypes.
How to Multiply in Python: A Guide to Use This Function
Jul 26, 2023 · In this section, we’ll create a Python program that multiplies two numbers. We’ll store these numbers in variables and use the multiplication operator to calculate the result. print(result) # Output: 63. Sometimes, we need to interact with …
How to multiply in Python - Altcademy Blog
Jun 13, 2023 · In Python, the most straightforward way to multiply two numbers is by using the * operator. This operator works with integers, floats, and even complex numbers. Here's a simple example: In this example, we define two variables, a and …
python - Creating a multiplying function - Stack Overflow
Mar 3, 2021 · def Multiply( num1, num2 ): answer = num1 * num2 return answer print(Multiply(2, 3)) The function Multiply will take two numbers as arguments, multiply them together, and return the results. I'm having it print the return value of the function when supplied with 2 and 3.