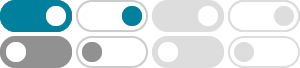
python - Numpy array dimensions - Stack Overflow
Jun 22, 2023 · You can use .ndim for dimension and .shape to know the exact dimension: >>> var = np.array([[1,2,3,4,5,6], [1,2,3,4,5,6]]) >>> var.ndim 2 >>> var.shape (2, 6) You can change the dimension using .reshape function: >>> var_ = var.reshape(3, …
Find the dimensions of a multidimensional Python array
Jul 8, 2013 · In Python, is it possible to write a function that returns the dimensions of a multidimensional array (given the assumption that the array's dimensions are not jagged)? For example, the dimensions of [[2,3], [4,2], [3,2]] would be [3, 2] , while the dimensions of [[[3,2], [4,5]],[[3,4],[2,3]]] would be [2,2,2] .
How can I find the dimensions of a matrix in Python?
Jan 23, 2018 · The correct pythonic way to get the dimensions of a matrix (formed using np.array) would be to use .shape. Let a be your matrix. To get the dimensions you would write a.shape , which would return (# columns, # rows).
NumPy: Get the number of dimensions, shape, and size of …
May 9, 2023 · You can get the number of dimensions, shape (length of each dimension), and size (total number of elements) of a NumPy array (numpy.ndarray) using the ndim, shape, and size attributes. The built-in len() function returns the size of the first dimension.
How to get the number of dimensions of a matrix using NumPy in Python …
Sep 30, 2022 · Use ndim attribute available with the NumPy array as numpy_array_name.ndim to get the number of dimensions. Alternatively, we can use the shape attribute to get the size of each dimension and then use len() function for the number of dimensions.
Python Shape Function: Find Dimensions of Arrays and …
Jul 6, 2021 · The shape function in Python yields a tuple that signifies the dimensions of a NumPy array or a Pandas DataFrame. In the case of a DataFrame, the tuple indicates the quantity of rows and columns. For a NumPy array, the tuple reveals …
How to Shape and Size of Array in Python - Delft Stack
Mar 4, 2025 · Learn how to determine the shape and size of arrays in Python using NumPy's shape() and size() functions. This article provides clear examples, detailed explanations, and practical insights to enhance your data manipulation skills in Python.
How to Get Array Dimension in Python [NumPy] - Srinimf
Oct 29, 2022 · Array shape. Below, you will find the dimension and size of the array. The first letter in (2,3) is ‘2.’ So it is two dimensional array. x = np.array([[1, 2, 3], [4, 5, 6]], np.int32) >>> type(x) <class 'numpy.ndarray'> >>> x.shape (2, 3) >>> x.dtype dtype('int32')
Python Numpy shape () - Get Array Dimensions | Vultr Docs
Nov 19, 2024 · In this article, you will learn how to employ the shape() function to ascertain the dimensions of arrays. Explore how to use this function in different scenarios such as checking the size of one-dimensional, two-dimensional, and higher-dimensional arrays. Import the NumPy library. Create a one-dimensional array.
How to find the length (or dimensions, size) of a NumPy matrix?
Oct 8, 2023 · Finding the length (or dimensions, size) of a NumPy matrix. Numpy provides us an efficient way called array.shape() which is a property of both NumPy array ndarray's and matrices.