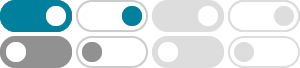
Get all unique values in a JavaScript array (remove duplicates)
With JavaScript 1.6 / ECMAScript 5 you can use the native filter method of an Array in the following way to get an array with unique values: function onlyUnique(value, index, array) { return array.indexOf(value) === index; } // usage example: var a = ['a', 1, 'a', 2, '1']; var unique = a.filter(onlyUnique); console.log(unique); // ['a', 1, 2, '1']
How to get distinct values from an array of objects in JavaScript?
Feb 28, 2013 · function uniqueBy(arr, fn) { var unique = {}; var distinct = []; arr.forEach(function (x) { var key = fn(x); if (!unique[key]) { distinct.push(key); unique[key] = true; } }); return distinct; } // usage uniqueBy(array, function(x){return x.age;}); // outputs [17, 35]
JavaScript – Unique Values (remove duplicates) in an Array
Nov 14, 2024 · There are various approaches to remove duplicate elements, that are discussed below. 1. Using set () – The Best Method. Convert the array into a Set in JS, which stores unique values, and convert it back to an array. 2. Using for Loop with includes () Method.
javascript - How to get unique values in an array - Stack Overflow
Mar 5, 2015 · Using jQuery, here's an Array unique function I made: Array.prototype.unique = function { var arr = this; return $.grep(arr, function (v, i) { return $.inArray(v, arr) === i; }); } console.log([1,2,3,1,2,3].unique()); // [1,2,3]
JavaScript – How To Get Distinct Values From an Array of Objects?
Nov 27, 2024 · Here are the different ways to get distinct values from an array of objects in JavaScript. 1. Using map() and filter() Methods. This approach is simple and effective for filtering distinct values from an array of objects. You can use map() to extract the property you want to check for uniqueness, and then apply filter() to keep only the first ...
How to Get Unique Values from an Array in JavaScript
Jun 23, 2023 · Discover easy techniques to get unique values from an array in JavaScript! Breakdown of methods, code snippets, and clear explanations for beginners and pros alike.
Getting All Unique Values (Remove All Duplicates) from Array in JavaScript
Jun 10, 2024 · Here are five ways to get all unique values (remove all duplicates) from an array in JavaScript: Use the “ […new Set (arr)]” expression, where you pass the array to the Set () constructor, and it will return the array with unique values. Output.
How to Get Unique Values in JavaScript Array - Ubiq BI
Feb 14, 2025 · Using spread operator and set function is the easiest way to get unique items in JavaScript array. If you want to selectively remove only some duplicates from your array, then you can use filter() function where you specify the exclusion of certain duplicates.
Top 4 Methods to Get Unique Values in a JavaScript Array
Nov 23, 2024 · Explore four effective methods to retrieve unique values from a JavaScript array while eliminating duplicates and maintaining order.
Get all unique values in a JavaScript array & remove duplicates
Jan 5, 2024 · Removing duplicates from an array in JavaScript can be done in a variety of ways, such as using Array.prototype.reduce(), Array.prototype.filter() or even a simple for loop. But there's a much simpler way to do it, using the built-in Set object.
- Some results have been removed