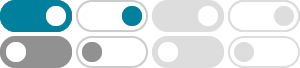
string - How to get the position of a character in Python ... - Stack ...
Feb 19, 2010 · Python has a in-built string method that does the work: index(). string.index(value, start, end) Where: Value: (Required) The value to search for. start: (Optional) Where to start the search. Default is 0. end: (Optional) Where to end the search. Default is to the end of the string. def character_index(): string = "Hello World!
python - Finding All Positions Of A Character In A String - Stack …
Sep 22, 2018 · I'm trying to find all the index numbers of a character in a python string using a very basic skill set. For example if I have the string "Apples are totally awesome" and I want to find the places where 'a' is in the string. My ideal output would be: 0 7 14 19 These are all the places in the string that an 'a' appears (I think)
python - find position of a substring in a string - Stack Overflow
May 13, 2012 · Here the best option is to use a regular expression. Python has the re module for working with regular expressions. We use a simple search to find the position of the "is": >>> match = re.search(r"[^a-zA-Z](is)[^a-zA-Z]", mystr) This returns the first match as a match object. We then simply use MatchObject.start() to get the starting position:
python - Find the nth occurrence of substring in a string - Stack …
This will find the second occurrence of substring in string. def find_2nd(string, substring): return string.find(substring, string.find(substring) + 1) Edit: I haven't thought much about the performance, but a quick recursion can help with finding the nth occurrence:
python - Find all the occurrences of a character in a string - Stack ...
Oct 22, 2012 · text.find() only returns the first result, and then you need to set the new starting position based on that. So like this: def findSectionOffsets(text): startingPos = 0 position = text.find("|", startingPos): while position > -1: print position startingPos = position + 1 position = text.find("|", startingPos)
python - Find index of last occurrence of a substring in a string ...
Apr 5, 2020 · Python String rindex() Method. Description Python string method rindex() returns the last index where the substring str is found, or raises an exception if no such index exists, optionally restricting the search to string[beg:end]. Syntax Following is the syntax for rindex() method −. str.rindex(str, beg=0 end=len(string)) Parameters
python - How to get char from string by index? - Stack Overflow
Jan 13, 2012 · Previous answers cover about ASCII character at a certain index. It is a little bit troublesome to get a Unicode character at a certain index in Python 2. E.g., with s = '한국中国にっぽん' which is <type 'str'>, __getitem__, e.g., s[i], does not lead you to where you desire. It will spit out semething like .
python - index of second repeated character in a string - Stack …
Jan 18, 2014 · I am trying a hangman code in python. For matching a character of a word , iam using index function to get the location of character. Ex :word = 'COMPUTER' user_input = raw_input('Enter a characte...
python - How to find all occurrences of a substring ... - Stack …
Python has string.find() and string.rfind() to get the index of a substring in a string. I'm wondering whether there is something like string.find_all() which can return all found indexes (not only the first from the beginning or the first from the end). For example:
python - Find a substring in a string and returning the index of the ...
There's a builtin method find on string objects. s = "Happy Birthday" s2 = "py" print(s.find(s2)) Python is a "batteries included language" there's code written to do most of what you want already (whatever you want).. unless this is homework :) find returns -1 if the string cannot be found.