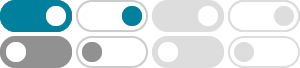
Create your Python Project Structure - Python in Plain English
Learn how to efficiently create multiple folders and files in Python using the os and pathlib modules. Enhance your project setup with these scripts.
Implementing an Interface in Python – Real Python
In this tutorial, you'll explore how to use a Python interface. You'll come to understand why interfaces are so useful and learn how to implement formal and informal interfaces in Python. You'll also examine the differences between Python …
oop - How do I implement interfaces in python? - Stack Overflow
Implementing interfaces with abstract base classes is much simpler in modern Python 3 and they serve a purpose as an interface contract for plug-in extensions. Create the interface/abstract base class:
Structuring Your Project — The Hitchhiker's Guide to Python
We need to consider how to best leverage Python’s features to create clean, effective code. In practical terms, “structure” means making clean code whose logic and dependencies are clear as well as how the files and folders are organized in the filesystem. Which functions should go into which modules? How does data flow through the project?
Build a Python Directory Tree Generator for the Command Line
Creating applications with a user-friendly command-line interface (CLI) is a useful skill for a Python developer. With this skill, you can create tools to automate and speed up tasks in your working environment. In this tutorial, you’ll build a Python …
Python Directory Tree Generator — Your File Hierarchy
May 28, 2024 · By leveraging Python’s `os` module and `tkinter` library, the project offers both a command-line and graphical user interface (GUI) for displaying directory trees.
console output - Creating a hierarchical file structure in Python …
May 21, 2023 · You could use pathlib for a lot of what you need. Given your list of paths and file sizes paths. def generate_file_structure(paths): for path_data in paths: path, size = path_data file_path = Path(path) file_dir = file_path.parent # Effectively run mkdir -p which creates any directories that don't exist file_dir.mkdir(exists_ok=True, parents=True)
GitHub - ghhrmnzdh/file-structure-generator: A Python script to ...
File Structure Generator is a lightweight Python utility that dynamically creates file and directory structures from user input. It is designed to help developers quickly set up organized project templates—supporting nested directories, CLI flexibility, and even AI-generated file structures.
Design a File System with Python. The problem is to create a file ...
Mar 28, 2023 · In this implementation, we have the insert_file function, which takes a file path and data as input, creates the directory (if it doesn't already exist), and then writes the data to the...
Simplify Folder Structures with a Python Directory Tree Generator
In this article, we will explore the concept behind a directory tree generator tool, how to structure a Python application layout, and how to use recursion, command-line interfaces, and pathlib to generate a directory tree.