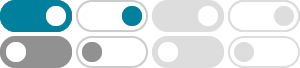
Python | Using 2D arrays/lists the right way - GeeksforGeeks
Jun 20, 2024 · A 2D array is essentially a list of lists, which represents a table-like structure with rows and columns. Creating a 1-D list. In Python, Initializing a collection of elements in a linear sequence requires creating a 1D array, which is a fundamental process. Although Python does not have a built-in data structure called a ‘1D array’, we can ...
python - Convert a 1D array to a 2D array in numpy - Stack Overflow
Sep 25, 2012 · convert a 1-dimensional array into a 2-dimensional array by adding new axis. b=a[:,np.newaxis]--it will convert it to two dimension. There is a simple way as well, we can use the reshape function in a different way: You can use flatten() from the numpy package. [3, 4], [5, 6]]) Output: [3 4] [5 6]] . Could you share your code?
python - Merging 1D arrays into a 2D array - Stack Overflow
Mar 16, 2018 · Is there a built-in function to join two 1D arrays into a 2D array? Consider an example: X=np.array([1,2]) y=np.array([3,4]) result=np.array([[1,3],[2,4]]) I can think of 2 simple solutions. The first one is pretty straightforward. np.transpose([X,y]) The other one employs a lambda function. np.array(list(map(lambda i: [a[i],b[i]], range(len(X)))))
Creating a one-dimensional NumPy array - GeeksforGeeks
Jan 27, 2025 · We can create a 1-D array in NumPy using the array () function, which converts a Python list or iterable object. Let’s explore various methods to Create one- dimensional Numpy Array. arrange () returns evenly spaced values within a given interval. Linspace () creates evenly space numerical elements between two given limits. Output: [ 3. 6.5 10.
Array creation — NumPy v2.2 Manual
NumPy arrays can be defined using Python sequences such as lists and tuples. Lists and tuples are defined using [...] and (...), respectively. Lists and tuples can define ndarray creation: a list of numbers will create a 1D array, a list of lists will create a 2D array, further nested lists will create higher-dimensional arrays.
python - Create a two-dimensional array with two one-dimensional arrays ...
How can I create a two-dimensional NumPy array effectively (like points mentioned above) with tp and fp? If you wish to combine two 10 element one-dimensional arrays into a two-dimensional array, np.vstack((tp, fp)).T will do it.
How to Create a 2D NumPy Array in Python? - Python Guides
Nov 20, 2023 · This tutorial explains how to create a 2D NumPy array in Python using six different methods like, array(), zeros(), ones(), full(), random(), and arange() with reshape() function. Skip to content Menu
Convert 1D array to 2D array in Python (numpy.ndarray, list)
May 15, 2023 · This article explains how to convert a one-dimensional array to a two-dimensional array in Python, both for NumPy arrays ndarray and for built-in lists list.
NumPy Creating Arrays - W3Schools
Create a 3-D array with two 2-D arrays, both containing two arrays with the values 1,2,3 and 4,5,6: import numpy as np arr = np.array([[[1, 2, 3], [4, 5, 6]], [[1, 2, 3], [4, 5, 6]]])
Convert a 1D array to a 2D Numpy array - GeeksforGeeks
Sep 8, 2022 · Convert a 1D array to a 2D Numpy array using numpy.reshape. Here, we are using np.reshape to convert a 1D array to 2 D array. You can divide the number of elements in your array by ncols.