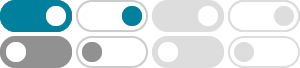
JavaScript: 6 Ways to Calculate the Sum of an Array
Feb 19, 2023 · This practical, succinct article walks you through three examples that use three different approaches to find the sum of all elements of a given array in Javascript (suppose this array only contains numbers). Without any further ado, let’s get started.
javascript - How to find the sum of an array of numbers - Stack Overflow
Dec 13, 2014 · function sumArray(array) { for ( var index = 0, // The iterator length = array.length, // Cache the array length sum = 0; // The total amount index < length; // The "for"-loop condition sum += array[index++] // Add number on each iteration ); return sum; }
Sum of an Array in JavaScript - GeeksforGeeks
6 days ago · The eval Function approach calculates the sum of an array by converting it to a string with elements joined by ‘+’, then using eval to evaluate the string as a JavaScript expression, returning the sum.
How to Find the Sum of an Array of Numbers in JavaScript
Nov 11, 2024 · To find the sum of an array of numbers in JavaScript, you can use several different methods. 1. Using Array reduce () Method. The array reduce () method can be used to find the sum of array. It executes the provided code for all the elements and returns a single output. Syntax. 2. Using for Loop.
JS Sum of an Array – How to Add the Numbers in a JavaScript Array
Mar 31, 2023 · In this article, you will learn how to calculate the sum of all the numbers in a given array using a few different approaches. Specifically, I will show you how to find the sum of all numbers in an array by using: A for loop; The forEach() method; The reduce() method; Let's get started! How to Calculate the Sum of an Array Using A for Loop in ...
Finding the sum of an array in javascript - Stack Overflow
Nov 12, 2015 · You can use Array.prototype.map and Array.prototype.reduce to make it easier: var ids = ['num_one', 'num_two', 'num_three']; var sum = ids .map(function(x) { return +document.getElementById(x).value; }) .reduce(function(a,b) { return a+b; }, 0); With this way, you will be able to introduce new elements into calculations with minimal changes.
How to Add the Numbers in a JavaScript Array - Expertbeacon
Aug 23, 2024 · In this comprehensive guide, you will learn several methods to calculate the sum of all the numbers in a given array using different approaches in JavaScript. Specifically, I will demonstrate how to find the total sum of all elements in an array by using: Let‘s explore each approach in detail.
javascript - Better way to sum a property value in an array
function sumOfArrayWithParameter (array, parameter) { let sum = null; if (array && array.length > 0 && typeof parameter === 'string') { sum = 0; for (let e of array) if (e && e.hasOwnProperty(parameter)) sum += e[parameter]; } return sum; }
How to Find the Sum of an Array of Numbers - W3docs
To find the sum of certain elements of an array in JavaScript, you can use a loop to iterate over the elements and add them up. Here's an example code that adds up the elements from indices 3-7 of an array:
How to Compute the Sum and Average of Elements in an Array in ...
Sep 2, 2024 · Computing the sum and average of elements in an array involves iterating through the array, accumulating the sum of its elements, and then dividing the sum by the total number of elements to get the average. This can be achieved using various techniques, from basic loops to more advanced functional methods provided by JavaScript.