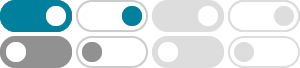
Searching Algorithms in Python - GeeksforGeeks
Feb 22, 2025 · In this tutorial, we've covered four fundamental searching algorithms in Python: Linear Search, Binary Search, Interpolation Search, and Jump Search. Each algorithm has its advantages and is suitable for different scenarios.
Searching Algorithms - GeeksforGeeks
Apr 13, 2025 · Searching algorithms are essential tools in computer science used to locate specific items within a collection of data. In this tutorial, we are mainly going to focus upon searching in an array.
A* Search Algorithm in Python - GeeksforGeeks
Apr 17, 2024 · Searching algorithms are fundamental techniques used to find an element or a value within a collection of data. In this tutorial, we'll explore some of the most commonly used searching algorithms in Python. These algorithms include Linear Search, Binary Search, Interpolation Search, and Jump Search.
A* Search Algorithm - GeeksforGeeks
Jul 30, 2024 · A* Search algorithm is one of the best and popular technique used in path-finding and graph traversals. Why A* Search Algorithm? Informally speaking, A* Search algorithms, unlike other traversal techniques, it has “brains”.
Linear Search Algorithm - GeeksforGeeks
Mar 27, 2025 · Linear Search finds the element in O(n) time, Jump Search takes O(n) time and Binary Search takes O(log n) time. The Interpolation Search is an improvement over Binary Se 15+ min read
Linear Search - Python - GeeksforGeeks
Apr 7, 2025 · The article explains how to find the index of the first occurrence of an element in an array using both iterative and recursive linear search methods, returning -1 if the element is not found.
Interpolation Search - Python - GeeksforGeeks
Feb 22, 2025 · The Interpolation search is a practical searching algorithm particularly for the uniformly distributed sorted arrays. It provides improvement over binary search by the efficiently estimating the probable position of the target element.
Introduction to Searching - Data Structure and Algorithm Tutorial
Oct 21, 2024 · Understanding the characteristics of searching in data structures and algorithms is crucial for designing efficient algorithms and making informed decisions about which searching technique to employ. Here, we explore key aspects and characteristics associated with searching:
Depth First Search or DFS for a Graph - Python - GeeksforGeeks
Feb 21, 2025 · Python Depth First Search Algorithm is used for traversing or searching tree or graph data structures. The algorithm starts at the root node (selecting some arbitrary node as the root node in the case of a graph) and explores as far …
Sorting Algorithms in Python - GeeksforGeeks
Apr 9, 2025 · Python has two main methods to sort a list, sort() and sorted(). Please refer Sort a list in Python for details. Sorting Techniques. The different implementations of sorting techniques in Python are: 1. Bubble Sort. Bubble Sort is the simplest sorting algorithm that works by repeatedly swapping the adjacent elements if they are in the wrong order.