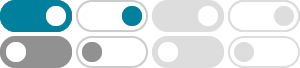
javascript - Remove duplicate values from JS array - Stack Overflow
Jan 25, 2016 · The most concise way to remove duplicates from an array using native javascript functions is to use a sequence like below: vals.sort().reduce(function(a, b){ if (b != a[0]) a.unshift(b); return a }, [])
4 Ways to Remove Duplicates from an Array in JavaScript
Mar 10, 2023 · Fortunately, in Javascript, there are some easy and surprisingly effective ways to remove duplicates from that array. The most common techniques are using the Set object, filter () method, for loop, and reduce () method.
Remove Duplicate Elements from JavaScript Array
Jan 9, 2025 · We can also use a third-party library such as Lodash or Underscore.js to remove duplicate elements from a Javascript array. The _.uniq() function returns the array which does not contain duplicate elements.
JavaScript Program to Remove Duplicates From Array
The for...of loop is used to loop through all the elements of an arr array. The indexOf() method returns -1 if the element is not in the array. Hence, during each iteration, if the element equals -1 , the element is added to uniqueArr using push() .
Remove duplicates in an array using forEach - Stack Overflow
Feb 19, 2019 · first initialize result with empty array. Then iterate through passed array, check if the index is its first occurance of item. push to result array. return result array. Alternative solution : function removeDup(arr) { let result = [] arr.forEach((item, index) => { if (result.indexOf(item) === -1) result.push(item) }); return result; }
How to Remove duplicate elements from array in JavaScript - GeeksforGeeks
May 29, 2024 · Given an array of numbers or strings containing some duplicate values, the task is to remove these duplicate values from the array without creating a new array or storing the duplicate values anywhere else.
JavaScript – Unique Values (remove duplicates) in an Array
Nov 14, 2024 · There are various approaches to remove duplicate elements, that are discussed below. 1. Using set () – The Best Method. Convert the array into a Set in JS, which stores unique values, and convert it back to an array. 2. Using for Loop with includes () Method.
Remove Duplicate Values From Array In JavaScript
May 27, 2024 · This tutorial will cover the different methods to remove duplicates from an Array, including using a for loop.
Remove Duplicate Elements from JavaScript Array - Intellipaat
Mar 28, 2025 · Method 5: Using a for() Loop to Remove Duplicates. Using for() loop also helps you to remove the duplicate values from an array. It can check each value of the original array with each value of the output array if the match is found …
How to remove duplicates from an array in JavaScript
Aug 25, 2021 · Here is one example of remove duplicates from an array in JavaScript using a for loop: const dedupeArray = someArray => { const history = { } const newDeduplicatedArray = [ ] for ( let i = 0 ; i < someArray . length ; i += 1 ) { if ( ! history ?.
- Some results have been removed