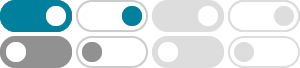
Find the function definition, function name, parameter(s), and return value. What is the “calling” function? What’s the difference between arguments and parameters? arguments are the values passed in when function is called! def main(): mid = average(10.6, 7.2) print(mid) Note that we’re storing the returned value in a variable!
Functional programming • Python supports functional programming idioms • Builtins for map, reduce, filter, closures, continuations, etc. • These are often used with lambda
It is important to understand, the scope of the variables used in functions. Lets look at an example. x=0 y=0 def incr(x): y=x+1 return y incr(5) print x, y Variables assigned in a function, including the arguments are called the local variables to the function. The variables defined in the top-level are called global variables.
There are three types of functions in Python: I. Built-in functions The Python interpreter has a number of functions built into it that are always available. They are listed here in alphabetical order. II. User-Defined Functions (UDFs): The Functions defined by User is known as User Defined Functions. These are defined with the keyword def III.
Write statement to call the function. Which Line Number Code will never execute? What will be the output of following code? What are the different types of actual arguments in function? Give example of any one of them.
print 'Hello' print 'Fun' There are two kinds of functions in Python. Built-in functions that are provided as part of Python - raw_input(), type(), float(), int() ... A function is some stored code that we use. A function takes some input and produces an output. A …
Guide To Python Print Function | PDF | Parameter (Computer Programming …
The document provides an in-depth guide to using the Python print function. It covers the basics of calling print and separating arguments.
To answer that Python has a print function and no printf function is only one side of the coin or half of the truth. One can go as far as to say that this answer is not true. So there is a "printf" in Python? No, but the functionality of the "ancient" printf is contained in Python.
for i in range(0, 500): print("I will not throw paper airplanes in class.") e.g., print, input, ... Why use functions? constants: literal values like 1, 3.14, "Hello, world!" big programs need teams, management, coordination, meetings, ...
Print Function : the print() function takes a list of values to print and writes them to the output, e.g., print('cat',5,'dog') print() # blank line print('pi is about', 3.14) Optional keyword arguments can be used to replace the defaults: space-character ( ' ' ) as a sep arator, the