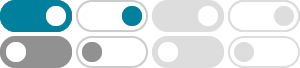
Python Print Star Patterns 14 Programs - EasyCodeBook.com
May 17, 2021 · Python Print Star Pattern Shapes – In this Python Programming tutorial, we will discuss printing different star patterns, shapes, pyramids, triangles, squares etc using nested for loops.
Program to construct the stars (*) pattern, using a nested for loop
Nov 22, 2024 · Use nested loops: Outer loop runs for the number of rows (i.e., size) First inner loop runs for the number of leading spaces (i.e., size - current row number - 1) Second inner loop runs for the number of stars (i.e., 2 * current row number + 1) Print the leading spaces, then print the stars with a space after each
Python Programs to Print Patterns – Print Number, Pyramid, Star ...
Sep 3, 2024 · Print star or number. Use the print() function in each iteration of nested for loop to display the symbol or number of a pattern (like a star (asterisk *) or number). Add a new line after each iteration of the outer loop. Add a new line using the print() function after each iteration of the outer loop so that the pattern displays appropriately
Star Pattern Programs in Python using For Loop
# Python Program to print star pattern using for loop def pattern(n): for i in range(n): for j in range(n): # printing stars print("* ",end="") print("\r") # take inputs n = int(input('Enter the number of rows: ')) # calling function pattern(n) Short Methods
creating a star pattern using an elif statement and for loop
Aug 29, 2023 · Using this we can do away with the inner loop and the extra call to print() row_count = 6 start = 1 end = (2 * row_count) + 1 for row_number in range(start, end): star_count = row_number if row_number <= row_count else end - row_number print("* " * star_count)
Print stars - one per line up to 5 using the for loop (python)
Sep 23, 2015 · def print_stars(count): for i in range(1, count): print('*' * i) print_stars(10) for i in range(1, count) will give 1, 2, 3, 4...count (count is a number like 10 ). And about print('*' * i) , first '*' is a string, that will be print.
Star Pattern in Python using For Loop-20 Programs - CSVeda
Aug 14, 2020 · Here are 20 codes to create star pattern in Python using Nested For loop. These codes will help you in understanding use of Python For Loop.
Printing Stars Patterns In Python. - Python in Plain English
Oct 19, 2023 · Today, In this article we are going to learn everything about printing star patterns using loops in Python. While learning any programming language, we encounter loops, and within loops, we come across printing patterns.
How to use `for` loop to print increasing star patterns in Python?
Let's explore how to use for loops to print increasing star patterns in Python. The simplest form of an increasing star pattern is a triangle of stars, where each row has one more star than the previous row. Here's an example: print('* ' * (i+1)) Explain Code. Practice Now. This will output: * * * * * Explain Code. Practice Now.
Star(*) or asterisk pattern in python using for loop - CodeSpeedy
Nested for loop is used in the program to make a star or asterisk pattern. Syntax: body of for. Output:- The outer loop gives i=0 in the first iteration and goes to the inner loop which will work for the range (0,6-i) and print the star (*) for 6 time in a line and the inner loop work is completed. After that it will come to next line by print ().