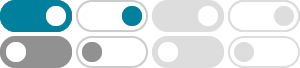
javascript - How to convert a plain object into an ES6 Map?
Apr 15, 2016 · With Object.entries, you can easily convert from Object to Map: const obj = { foo: 'bar', baz: 42 }; const map = new Map(Object.entries(obj)); console.log(map); // Map { foo: "bar", baz: 42 } Share
javascript - map function for objects (instead of arrays ... - Stack ...
Feb 11, 2013 · // Takes the object to map and a function from (key, value) to mapped value. const mapObject = (obj, fn) => { const newObj = {}; Object.keys(obj).forEach(k => { newObj[k] = fn(k, obj[k]); }); return newObj; }; // Maps an object to a new …
How to Use the JavaScript Map and Set Objects – Explained with …
Feb 21, 2024 · Map and Set are two JavaScript data structures you can use to store a collection of values, similar to Objects and Arrays. They are specialized data structures that can help you store and manipulate related values. In this tutorial, we will see how Map and Set work in detail and when to use them.
Map and Set - The Modern JavaScript Tutorial
Map is a collection of keyed data items, just like an Object. But the main difference is that Map allows keys of any type. Methods and properties are: new Map() – creates the map. map.set(key, value) – stores the value by the key. map.get(key) – returns the value by the key, undefined if key doesn’t exist in map.
Map.prototype.set() - JavaScript | MDN - MDN Web Docs
Mar 6, 2025 · The set() method of Map instances adds or updates an entry in this map with a specified key and a value.
JavaScript Map set() Method - W3Schools
The set() method can also be used to change existing Map values: The set() method adds an element to a map. The set() method updates an element in a map. Required. The element key. Required. The element value. The map object. map.set() is an ECMAScript6 (ES6) feature. ES6 (JavaScript 2015) is supported in all modern browsers since June 2017:
javascript - How to convert Map to array of object ... - Stack Overflow
Jun 27, 2019 · const map = new Map().set('a', 1).set('b', 2).set('c', 3) const mapArr = [...map] // array of arrays Map each subarray as key-value pairs. map function returns a new array containing object of key-value pairs. const arr = mapArr.map(([key, value]) => ({key, value}))
How to Use JavaScript Collections – Map and Set
Oct 5, 2020 · To add value to a Map, use the set(key, value) method. The set(key, value) method takes two parameters, key and value, where the key and value can be of any type, a primitive (boolean, string, number, etc.) or an object: Output: Please note, if you use the same key to add a value to a Map multiple times, it'll always replace the previous value:
JavaScript Map set() Method - GeeksforGeeks
Jul 15, 2024 · The JavaScript map.set() method is used to add key-value pairs to a Map object. It can also be used to update the value of an existing key. Each value must have a unique key so that they get mapped correctly. Syntax: GFGmap.set(key, value); Parameters: This method accepts two parameters as mentioned above and described below:
JavaScript Map Object - JavaScript Tutorial
In this tutorial, you have learned how to work with the JavaScript Map object and its useful methods to manipulate entries in the map.
- Some results have been removed