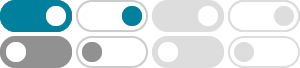
Find the min/max element of an array in JavaScript
Or with the new spread operator, getting the maximum of an array becomes a lot easier. var arr = [1, 2, 3]; var max = Math.max(...arr); Maximum size of an array. According to MDN the apply …
Finding the max value of a property in an array of objects
Nov 22, 2023 · FWIW my understanding is when you call apply on a function it executes the function with a specified value for this and a series of arguments specified as an array. The …
javascript - Return index of greatest value in an array - Stack …
Jul 11, 2013 · To find the index of the greatest value in an array, copy the original array into the new array and then sort the original array in decreasing order to get the output [22, 21, 7, 0]; …
javascript - Get the element with the highest occurrence in an …
Nov 4, 2016 · Based on Emissary's ES6+ answer, you could use Array.prototype.reduce to do your comparison (as opposed to sorting, popping and potentially mutating your array), which I …
max of array in JavaScript - Stack Overflow
Jul 16, 2017 · Given an array of values [1,2,0,-5,8,3], how can I get the maximum value in JavaScript? I know I can write a loop and keep track of the maximum value, but am looking for …
Find the Max Value in an array using JavaScript function
Line one. max function is passed the array [4,12,3,8,0,22,56], Line two. max variable is set to the first item in the array. max is 4, Line three. A for loop is started which will start from 1 and keep …
javascript - Getting key with the highest value from object - Stack ...
Dec 9, 2014 · This returns an array, with the keys for all of them with the maximum value, in case there are some that have equal values. For example: if . const obj = {apples: 1, bananas: 1, …
Fast way to get the min/max values among properties of object
Jun 21, 2012 · Is there a fast way to get the minimum and maximum value among the properties without having to loop through them all? because the object I have is huge and I need to get …
javascript - Getting max value(s) in JSON array - Stack Overflow
Apr 8, 2014 · I'm trying to create a JavaScript function which takes information from an array in an external JSON and then takes the max value (or the top 5 values) for one of the JSON …
How to return the object with highest value from an array
You can use Array#map and Array#find // First, get the max vote from the array of objects var maxVotes = Math.max(...games.map(e => e.votes)); // Get the object having votes as max …