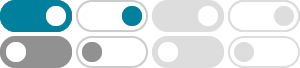
How to inverse a matrix using NumPy - GeeksforGeeks
May 5, 2023 · Inverse Matrix using NumPy. Python provides a very easy method to calculate the inverse of a matrix. The function numpy.linalg.inv() is available in the NumPy module and is used to compute the inverse matrix in Python. Syntax: numpy.linalg.inv(a) Parameters: a: Matrix to be inverted; Returns: Inverse of the matrix a.
python - Inverse of a matrix using numpy - Stack Overflow
You can use numpy.linalg.inv to invert arrays: inverse = numpy.linalg.inv(x) Note that the way you're generating matrices, not all of them will be invertible. You will either need to change the way you're generating matrices, or skip the ones that aren't invertible. try: inverse = numpy.linalg.inv(x) except numpy.linalg.LinAlgError: # Not ...
algorithm - Python Inverse of a Matrix - Stack Overflow
Jul 3, 2013 · Here is an example of how to invert a matrix, and do other matrix manipulation. from numpy import matrix from numpy import linalg A = matrix( [[1,2,3],[11,12,13],[21,22,23]]) # Creates a matrix. x = matrix( [[1],[2],[3]] ) # Creates a matrix (like a column vector). y = matrix( [[1,2,3]] ) # Creates a matrix (like a row vector). print A.T ...
Compute the inverse of a matrix using NumPy - GeeksforGeeks
Feb 26, 2021 · Using determinant and adjoint, we can easily find the inverse of a square matrix using below formula, A -1 = adj(A)/det(A) "Inverse doesn't exist" . We can find out the inverse of any square matrix with the function numpy.linalg.inv (array). Returns: Inverse of the matrix a. Example 1: Output: [ 1.25 -0.25]] [-0.125 0.25 -0.125 ]
Calculating the Inverse of a Matrix using Python - scicoding.com
Feb 20, 2023 · Instead, you can use scipy.linalg.inv to calculate matrix inversion. The interface for scipy.linalg.inv is exactly the same as for the NumPy counterpart. You just pass the matrix to invert and get the inverted matrix as a return. Again, what this should return is the identity matrix.
An In-Depth Guide to Computing the Inverse Matrix in NumPy
Dec 27, 2023 · NumPy provides an efficient numpy.linalg.inv() function for calculating the inverse of a matrix in Python. In this comprehensive guide, we will explore all aspects of computing inverse matrices using NumPy in detail.
NumPy Inverse Matrix in Python - Spark By Examples
Mar 27, 2024 · NumPy linalg.inv() function in Python is used to compute the (multiplicative) inverse of a matrix. The inverse of a matrix is that matrix which when multiplied with the original matrix, results in an identity matrix. In this article, I will explain how to use the NumPy inverse matrix to compute the inverse of the matrix array using this function.
Invert a Matrix or Numpy Array in Python - Online Tutorials Library
Use the linalg.inv() function(calculates the inverse of a matrix) of the numpy module to calculate the inverse of an input 3x3 matrix by passing the input matrix as an argument to it and print the inverse matrix.
How to Find Inverse of a Matrix Using Numpy – allinpython.com
In this post, we will learn how to find inverse of a matrix using numpy with detailed exaplanation and example. Mathematically, the inverse of a matrix is only possible if it satisfies the following conditions: The determinant of the matrix should be non-zero (det (A) ≠ 0), or you can say the matrix is non-singular.
Understanding the Inverse of a Matrix in NumPy - Medium
Jan 29, 2025 · Now that we know how to check whether a matrix is invertible, let’s dive into actually finding the inverse using NumPy. The function numpy.linalg.inv() is all we need to do this. Let’s...