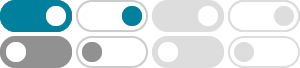
iteration - How to loop backwards in python? - Stack Overflow
def reverse(text): reversed = '' for i in range(len(text)-1, -1, -1): reversed += text[i] return reversed print("reverse({}): {}".format("abcd", reverse("abcd")))
Backward iteration in Python - GeeksforGeeks
Nov 20, 2024 · Python provides various methods for backward iteration, such as using negative indexing or employing built-in functions like reversed(). Use reversed () method when we simply need to loop backwards without modifying original sequence or there is …
python - How do I reverse a list or loop over it backwards?
Oct 15, 2010 · Reverse an existing list in-place (altering the original list variable) Best solution is object.reverse() method; Create an iterator of the reversed list (because you are going to feed it to a for-loop, a generator, etc.) Best solution is reversed(object) which creates the iterator
loops - Traverse a list in reverse order in Python - Stack Overflow
Feb 10, 2009 · How do I traverse a list in reverse order in Python? So I can start from collection[len(collection)-1] and end in collection[0]. I also want to be able to access the loop index.
Reversing a List in Python - GeeksforGeeks
Oct 15, 2024 · If we want to reverse a list manually, we can use a loop (for loop) to build a new reversed list. This method is less efficient due to repeated insertions and extra space required to store the reversed list.
Reverse an Array upto a Given Position - GeeksforGeeks
Jan 20, 2025 · reversed () is an efficient technique for modifying a list directly, without creating any extra copies of the list. This approach modifies the original array in-place, avoiding the need for additional space, which makes it both time and space efficient. Explanation:
Python For Loop in Backwards - Spark By Examples
May 30, 2024 · Usually, Python for loop is implemented in a forward direction however sometimes you would be required to implement for loop in the backward direction. You can easily achieve this by using reversed () function and the range() function, with the negative step.
5 Methods to Reverse Array in Python (reverse, recursion etc)
Sep 23, 2022 · Learn how to reverse an array in python using the 5 best methods. This includes reverse(), recursion, swapping, and slicing.
Reverse an Array in Python
Jun 28, 2023 · Reversing an array in Python is a fundamental operation that can be performed in multiple ways, including slicing, using built-in functions, and in-place reversal with loops. Understanding these methods provides flexibility in handling …
PythonHaven | Reversing an Array in Python
The provided Python code snippet demonstrates how to reverse an array and output the result. The approach here involves using a for loop to traverse the array in reverse order and store the elements in a new variable.