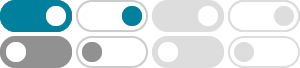
Reverse an array in Java - GeeksforGeeks
Nov 25, 2024 · The simplest way to reverse an array in Java is by using a loop to swap elements or by using the helper methods from the Collections and Arrays classes. Example: Let’s take an example to reverse an array using a basic loop approach.
How do I reverse an int array in Java? - Stack Overflow
Jan 26, 2010 · To reverse an int array, you swap items up until you reach the midpoint, like this: int temp = validData[i]; validData[i] = validData[validData.length - i - 1]; validData[validData.length - i - 1] = temp; The way you are doing it, you swap each element twice, so the result is …
5 Best Ways to Reverse an Array in Java - Codingface
May 20, 2022 · We can reverse the order of an Array in Java by following methods: 1. Reverse an Array by using a simple for loop through iteration. 2. Reverse an Array by using the in-place method. 3. Reverse an Array by using ArrayList and Collections.reverse( ) method 4. Reverse an Array by using StringBuilder.append( ) method 5.
Array Reverse – Complete Tutorial - GeeksforGeeks
Sep 25, 2024 · // Java Program to reverse an array using Recursion import java.util.Arrays; class GfG {// recursive function to reverse an array from l to r static void reverseArrayRec (int [] arr, int l, int r) {if (l >= r) return; // Swap the elements at the ends int temp = arr [l]; arr [l] = arr [r]; arr [r] = temp; // Recur for the remaining array ...
reversing an array of characters without creating a new array
Nov 8, 2009 · Reverse an array of characters without creating a new array using java. int middle = array.length / 2; String temp; int j = array.length -1; for (int i = 0 ; i < middle; i++) { temp = array[i]; array[i] = array[j]; array[j] = temp; j--; System.out.println(Arrays.toString(array));
Reverse An Array In Java - 3 Methods With Examples - Software …
Apr 1, 2025 · Q #1) How do you Reverse an Array in Java? Answer: There are three methods to reverse an array in Java. Using a for loop to traverse the array and copy the elements in another array in reverse order. Using in-place reversal in which the elements are swapped to place them in reverse order.
How to Invert an Array in Java - Baeldung
Jan 8, 2024 · In this quick article, we’ll show how we can invert an array in Java. We’ll see a few different ways to do this using pure Java 8-based solutions – some of those mutate an existing array and some create a new one. Next, we’ll look at two solutions using external libraries — one using Apache Commons Lang and one using Google Guava. 2.
Reversing an Array in Java - Stack Overflow
Apr 9, 2011 · If you want to reverse the array in-place: Collections.reverse(Arrays.asList(array)); It works since Arrays.asList returns a write-through proxy to the original array.
How to Reverse an Array in Java: Step-by-Step Guide
Feb 14, 2025 · For example, the following method returns an array that is the reversal of another array. public static int [] revers(int [] list){ int[] result = new int[list.length]; for (int i=0, j=result.length-1; i<list.length; i++, j--){ result[j]= list[i]; } return result; }
Reverse an Array in Java - Tpoint Tech
In this tutorial, we will discuss how one can reverse an array in Java. In the input, an integer array is given, and the task is to reverse the input array.