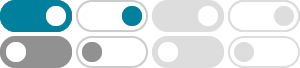
Python Classes without using def __init__ (self) - Stack Overflow
Your code is fine. The example below shows a minimal example. You can still instantiate a class that doesn't specify the __init__ method. Leaving it out does not make your class abstract. class A: def a(self, a): print(a) ob = A() ob.a("Hello World")
How do I use a class method without passing an instance to it?
Nov 26, 2024 · To achieve this, you wouldn't need to put them in a class. Just move them to a module and leave them at module level. Ways of exposing methods in a python module: module foo.py: return "I am a module method" @staticmethod. def static_method(): # the static method gets passed nothing. return "I am a static method" @classmethod.
python - Creating an instance of a class without defining the …
Dec 9, 2017 · First example - with the init method: class dog: def __init__(self,name): self.name=name print('My name is',name) Bob = dog('Bob') Second example - without the init method: class dog: def init_instance(self,name): self.name = name …
How to Call a Method on a Class Without Instantiating it in Python ...
Feb 23, 2024 · In this article, we'll dive into four different ways to do just that in Python. Below are the possible approaches to using a class and methods without instantiating it in Python. In the below approach code, the MathOperations class utilizes …
classmethod() in Python - GeeksforGeeks
Sep 5, 2024 · In Python, the classmethod () function is used to define a method that is bound to the class and not the instance of the class. This means that it can be called on the class itself rather than on instances of the class. Class methods are useful when you need to work with the class itself rather than any particular instance of it.
Python Classes and Objects - W3Schools
Python Classes/Objects. Python is an object oriented programming language. Almost everything in Python is an object, with its properties and methods. A Class is like an object constructor, or a "blueprint" for creating objects.
Calling Methods in Python 3 without ‘self’ Keyword: A Guide
Jul 11, 2024 · When calling a method in Python without using the ‘self’ keyword, we need to use the class name followed by the method name. Here is an example: class MyClass: def say_hello(): print("Hello, world!")
9. Classes — Python 3.13.3 documentation
1 day ago · Python classes provide all the standard features of Object Oriented Programming: the class inheritance mechanism allows multiple base classes, a derived class can override any methods of its base class or classes, and a method can call the method of a base class with the same name. Objects can contain arbitrary amounts and kinds of data.
Instantiating a Class in Python 3 Without Calling __init__()
Apr 6, 2024 · Overall, these examples demonstrate different ways to instantiate a class in Python without calling the __init__() method. Whether it is using object.__new__(), type.__call__(), or a custom metaclass, these techniques provide flexibility in object creation and initialization.
Classes with attributes but no methods - Stack Overflow
Oct 6, 2018 · Instead of creating classes like that though, I highly suggest you to use dataclasses for this type of behavior. Another option, for immutable types is to use namedtuples. That's good for readability, and there is no problem in defining …