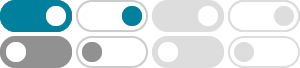
How to sort an array in a single loop? - GeeksforGeeks
Jun 11, 2021 · Given an array of size N, the task is to sort this array using a single loop. How the array is sorted usually? There are many ways by which the array can be sorted in ascending order, like: Selection Sort; Binary Sort; Merge Sort; Radix Sort; Insertion Sort, etc; In any of these methods, more than 1 loops is used. Can the array the sorted using ...
Using a For Loop to Manually Sort an Array - Java
Jan 12, 2016 · I'm having trouble manually sorting the array with a for loop. It works except for the first and last number. Here's my code: for(int j = 0; j < numbers.length - 1; j++) if(numbers[i] < numbers[j + 1]) tempVar = numbers [j + 1]; numbers [j + 1]= numbers [i]; numbers [i] = tempVar;
How to sort an array in a single loop? - Stack Overflow
Aug 12, 2015 · Sorting an array using java in Single Loop: public int[] getSortedArrayInOneLoop(int[] arr) { int temp; int j; for (int i = 1; i < arr.length; i++) { j = i - 1; if (arr[i] < arr[j]) { temp = arr[j]; arr[j] = arr[i]; arr[i] = temp; i = 1; } } return arr; }
Java program to sort a one dimensional array in ascending order
Dec 23, 2023 · Here, we are implementing a java program to sort an array (one-dimensional array) in ascending order? We are reading array elements, arranging them in ascending order and printing the sorted array.
One Dimensional Array in Java - GeeksforGeeks
May 15, 2024 · In Java, looping through an array or Iterating over arrays means accessing the elements of the array one by one. We have multiple ways to loop through an array in Java. Example 1: Here, we are using the most simple method i.e. using for …
how to sort a string or array of number using one loop
Jun 26, 2015 · The code is not more efficient than doing it in a double loop (it's still O(n^2)), and using a smart sorting algorithm (including the one already exists in java: Arrays.sort()), will do it more efficiently and more easily.
Sorting in Java - GeeksforGeeks
Mar 15, 2024 · So there is sorting done with the help of brute force in java with the help of loops and there are two in-built methods to sort in Java. Ways of sorting in Java. Let us discuss all four of them and propose a code for each one of them. Way 1: Using loops. Way 2: Using sort () method of Arrays class.
Java How To Sort an Array - W3Schools
You can use the sort() method, found in java.util.Arrays, to sort an array: Example import java.util.Arrays; public class Main { public static void main(String[] args) { String[] cars = {"Volvo", "BMW", "Tesla", "Ford", "Fiat", "Mazda", "Audi"}; Arrays.sort(cars); for (String i : cars) { System.out.println(i); } } }
How to Sort an Array in Java - Tpoint Tech
In this section, we will learn how to sort array in Java in ascending and descending order using the sort() method and without using the sort() method. Along with this, we will also learn how to sort subarray in Java .
7 Examples to Sort One and Two Dimensional String and Integer Array …
Aug 4, 2014 · You can use Arrays.sort () method to sort both primitive and object array in Java. But, before using this method, let's learn how the Arrays.sort () method works in Java. This will not only help you to get familiar with the API but also its inner workings.